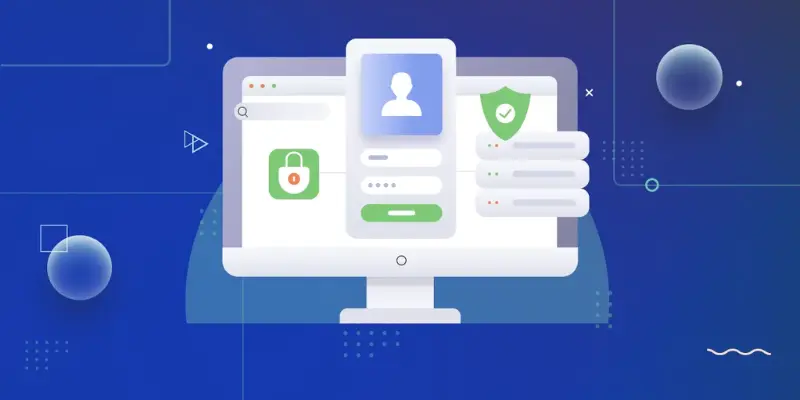
User authentication is the cornerstone of any secure web application. Whether you’re building an e-commerce platform, a SaaS product, or a personal blog with member access, you need a secure login system that protects sensitive user data. Node.js, with its asynchronous architecture and robust package ecosystem, makes it easier to build scalable and secure backend systems. In this guide, we’ll explore how to build a secure login system using Node.js and other modern tools—without diving into code—focusing on best practices, security layers, and key implementation strategies. If you’re looking to gain hands-on skills, enrolling in a Full Stack Course in Pune can be an excellent start.
Why Node.js is Ideal for Authentication Systems
Node.js is a JavaScript runtime built on Chrome’s V8 engine. It enables fast, event-driven server-side development and is highly favored for building APIs and backend services. When it comes to authentication, Node.js is highly compatible with tools like:
- Express.js for routing and middleware support
- Bcrypt.js for secure password hashing
- Passport.js for user authentication strategies
- JWT (JSON Web Token) for token-based authentication
- Express-session for session handling
Core Components of a Secure Login System
Let’s break down the essential parts of a login system, and how each contributes to security:
1. Secure User Registration
Before login comes user registration. Collect only necessary information (email, username, password) and validate it thoroughly. Use a library like express-validator to prevent injection attacks by checking for data type, format, and length.
- Ensure strong password requirements (e.g., mix of letters, numbers, symbols).
- Hash passwords using Bcrypt with proper salting before storing them in the database.
- Avoid sending plain-text passwords via email or storing them anywhere outside the secure database.
Joining a Full Stack Developer Course in Gurgaon will expose you to hands-on labs that teach these essential registration and validation techniques.
2. Password Hashing and Storage
Storing raw passwords is a major security risk. Store the hashed password after hashing it with Bcrypt. Salting the password adds an extra layer of protection by making it unique—even if two users use the same password.
Benefits of using Bcrypt:
- It’s computationally expensive, slowing down brute-force attacks.
- It auto-generates salts.
- It’s widely used and tested in production environments.
3. Login Authentication Methods
There are two main approaches:
- Session-Based Authentication: Stores user info on the server and uses a session cookie to track users. Good for traditional web apps.
- Token-Based Authentication (JWT): Sends an encrypted token to the client after login, which is used to access protected routes. Best for modern single-page apps (SPAs) and mobile apps.
4. Input Validation and Rate Limiting
Input validation prevents malicious data from entering your system. Always validate login and registration fields on both client and server sides.
Add rate limiting using tools like express-rate-limit to prevent brute-force attacks. This middleware limits the number of login attempts a user can make in a set timeframe.
You may learn how to incorporate these security layers into an actual application stack by enrolling in courses like the Full Stack Developer Course in Hyderabad.
5. HTTPS and Secure Cookies
Using HTTPS ensures that all data between client and server is encrypted. It’s non-negotiable for any app handling login credentials.
When dealing with cookies (especially in session-based logins), always set:
- HttpOnly: Prevents JavaScript from accessing cookies
- Secure: Ensures cookies are sent only over HTTPS
- SameSite: Prevents CSRF (Cross-Site Request Forgery)
6. Two-Factor Authentication (Optional but Recommended)
For added security, implement two-factor authentication (2FA). You can employ authenticator applications or send a one-time password (OTP) to the user’s email or mobile device.
Node packages like speakeasy and otplib can help implement this feature effectively. These tools are taught in courses like the Full Stack Developer Course in Mumbai, helping learners create real-world, enterprise-grade systems.
Logging and Monitoring
In a secure system, you should also implement logging mechanisms. Track failed login attempts, unusual access times, and geographic anomalies. Tools like Winston or cloud monitoring platforms like Datadog and Loggly can help keep tabs on suspicious behavior.
Building a secure login system in Node.js is a multi-layered process that requires thoughtful planning and implementation. From password hashing and data validation to HTTPS protocols and token management, every detail plays a role in keeping user data safe.
As cyber threats evolve, so must your login security measures. Keep your libraries updated, audit your code regularly, and always prioritize secure development practices. By leveraging Node.js and its ecosystem of authentication tools, you can build a login system that not only performs well but also protects your users every step of the way.
Also Check: Exploring the Role of APIs in Full-Stack Development